RestAPI讀取溫濕度
# PinToo之RestAPI讀取溫濕度
# 1. 說明
範例採用RestAPI通訊,來讀取連線到NodeMCU的DHT22溫濕度感測器的數據。NodeMCU是以ESP8266(ESP12)晶片為基礎,包含了WiFi,GPIO,PWM,ADC,I2C等功能的主控板,執行效率高,非常適合物聯網應用開發,因為它內建了WiFi功能,與Arduino相容,Arduino中可用的感測器基本都可用於NodeMCU。範例中使用的DHT22感測器正極(長腳)連線接至NodeMCU Vin針腳,負極連線至NodeMCU GND針腳,訊號針腳連線至NodeMCU D5針腳。
範例中使用的NodeMCU的ESP8266無線網路,先從路由器獲取IP,連線網路成功后,啟動Rest服務,通過訪問對應的地址獲取溫濕度數據。
通過範例學習,可以掌握Rest通訊的基本通訊原理,並結合NodeMCU開發板進行LED的控制功能。
# 2. 零件連線圖
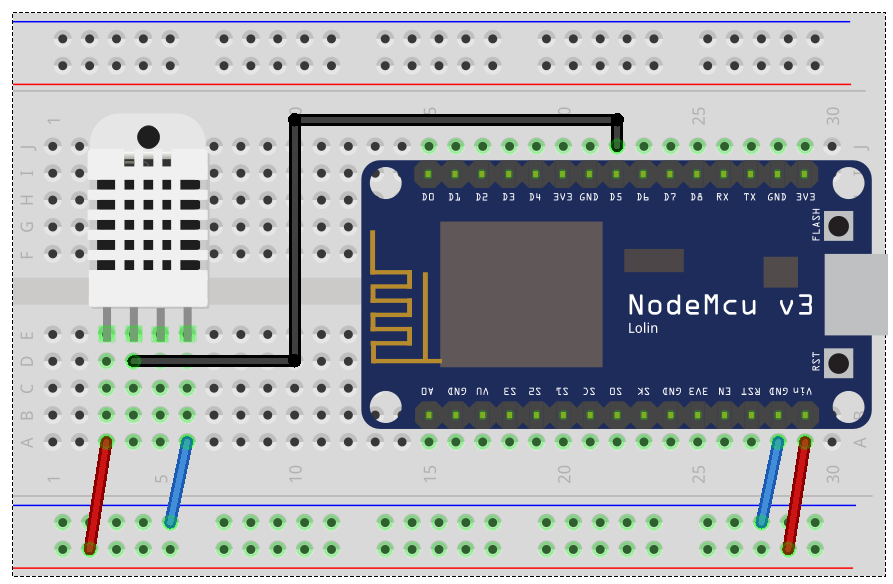
# 3. 使用零件
序 | 零件名稱 | 數量 |
---|---|---|
1 | NodeMCU開發板 | 1 |
2 | USB數據線 | 1 |
3 | 麵包板 | 1 |
4 | 杜邦線 | 若干 |
6 | DHT22溫濕度感測器 | 1 |
# 4. Arduino流程圖
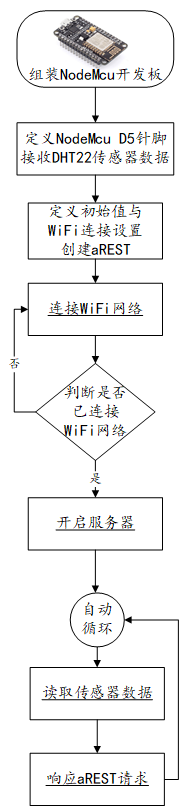
# 5. Arduino程式
使用Arduino IDE 編譯並上傳以下Arduino程式。
// 使用 NodeMCU 開發板
// aREST
//https://github.com/marcoschwartz/aREST
//aRESTUI
//https://github.com/marcoschwartz/aREST_UI
//DHT Library
//https://github.com/adafruit/DHT-sensor-library
#include <ESP8266WiFi.h>
#include <aREST.h>
#include <aREST_UI.h>
#include <DHT.h>
#define DHT_PIN 14 //定義LED針腳D5
#define DHTTYPE DHT22 // 定義溫濕度感測器型別 DHT22
DHT dht(DHT_PIN, DHTTYPE);//初始化感測器
// 建立aREST實體
aREST_UI rest = aREST_UI();
// WiFi連線參數
const char* ssid = "WIFI_SSID";
const char* password = "WIFI_PASSWORD";
// 監聽TCP連線
#define LISTEN_PORT 80
// 建立WiFi伺服器
WiFiServer server(LISTEN_PORT);
// 定義傳遞給API的變數
float temperature;
float humidity;
void setup(void) {
// 開啟通訊埠
Serial.begin(9600);
// 設定標題
rest.title("aREST DHT Sensor");
// 初始化 DHT
dht.begin();
// 初始化變數,並將其傳遞給REST API
rest.variable("temperature", &temperature);
rest.variable("humidity", &humidity);
// 設定標籤
rest.label("temperature");
rest.label("humidity");
// Give name and ID to device
rest.set_id("1");
rest.set_name("esp8266");
// 給設備定義ID與名稱
rest.set_id("1");
rest.set_name("esp8266");
//指定IP位址,請自行在此加入WiFi.config()敘述。
WiFi.config(IPAddress(192,168,0,171), // IP地址
IPAddress(192,168,0,1), // 閘道器地址
IPAddress(255,255,255,0)); // 子網掩碼
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
// 啟動伺服器
server.begin();
Serial.println("Server started");
// 輸出IP地址
Serial.println(WiFi.localIP());
}
void loop() {
//讀取溫濕度
humidity = dht.readHumidity();
temperature = dht.readTemperature();
// 響應aREST
WiFiClient client = server.available();
if (!client) {
return;
}
while (!client.available()) {
delay(1);
}
rest.handle(client);
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
# 6. 設計明細
開啟PinToo設計器,分別加入下插圖之控制元件。或者點選左上角的[打開模板Lib檔案]
選擇模板檔案來打開對應模板。
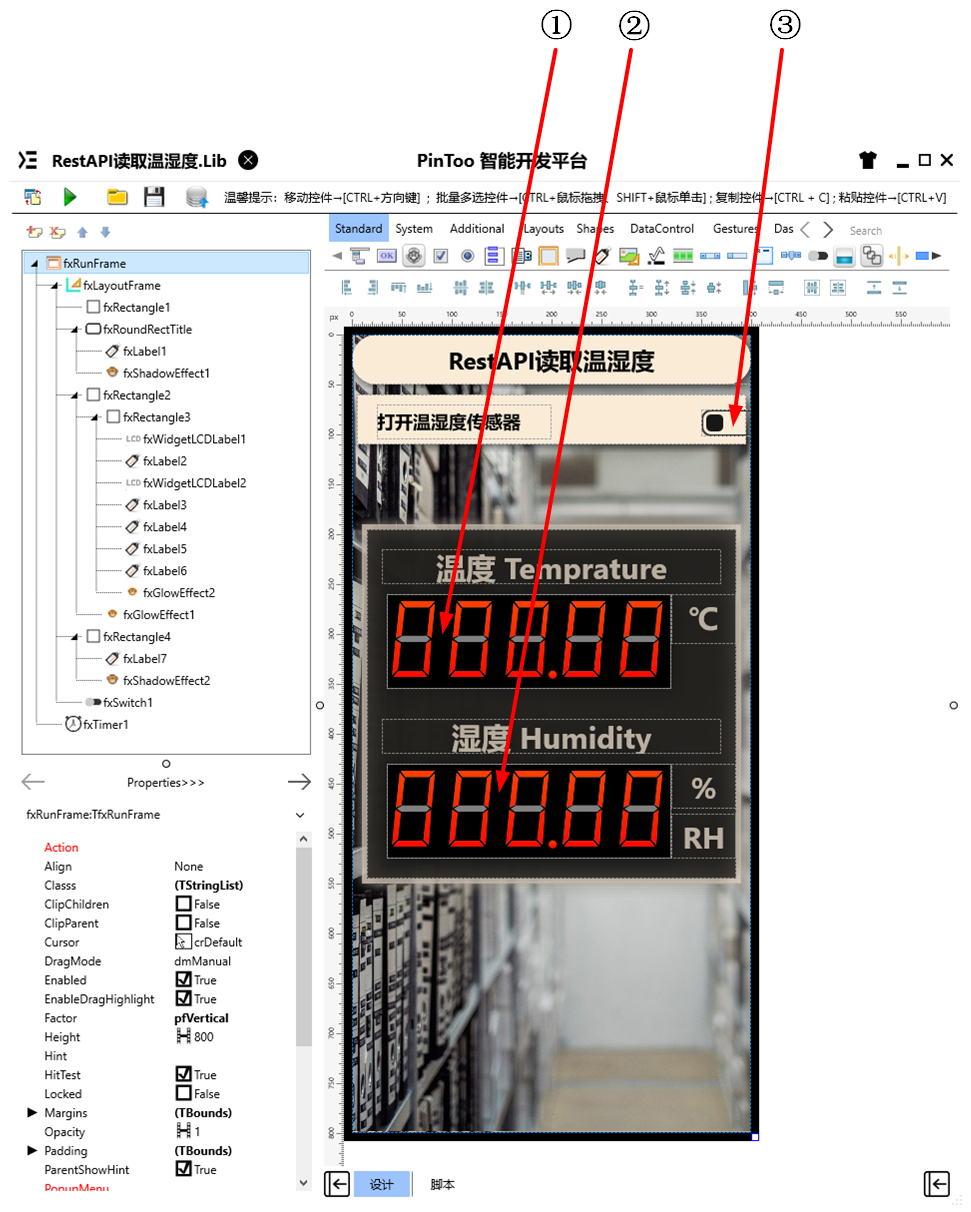
①:TfxWidgetLCDLabel元件,控制元件名稱為fxWidgetLCDLabel1
。
②:TfxWidgetLCDLabel元件,控制元件名稱為fxWidgetLCDLabel2
。
③:TfxSwitch元件,控制元件名稱為fxSwitch1
。
fxRunFrame屬性設定
Height
:設定頁面高度=800
。Width
:設定頁面寬度=400
。
fxTimer1屬性設定
Interval
:設定計時器觸發的時間間隔,單位為ms,設定為5000
。Enabled
:設定是否啟用,設定為False
。
①fxWidgetLCDLabel1屬性設定
Height
:設定控制元件高度=95
。Width
:設定控制元件寬度=285
。Color
:設定背景顏色=Black
。
②fxWidgetLCDLabel2屬性設定
Height
:設定控制元件高度=95
。Width
:設定控制元件寬度=285
。Color
:設定背景顏色=Black
。
# 7. 程式設計
點選程式設計界面右下角的按鈕,切換至單元選擇界面,勾選需要使用的單元。該程式需要引用ufxFunctions
、JSON
單元。
# 7.1. 程式初始設定
該程式無初始設定。
# 7.2. 事件設定
- fxTimer1-OnTimer事件
計時器定時觸發,每隔五秒讀取溫濕度數據並顯示。
Procedure fxTimer1OnTimer(Sender: TObject);
//計時器定時讀取溫濕度
var
hum :String;
tmp :String;
JSONStringHUM: String;
JSONStringTMP: String;
J: TJSONObject;
ValueHUM: TJSONValue;
ValueTMP: TJSONValue;
Begin
J := TJSONObject.Create;
Try
JSONStringTMP := fxfun.UrlDecode(fxfun.NetHttpGet('http://192.168.0.171/temperature'));
JSONStringHUM := fxfun.UrlDecode(fxfun.NetHttpGet('http://192.168.0.171/humidity'));
ValueTMP := fxfun.ParseJSONObject(JSONStringTMP);
ValueHUM := fxfun.ParseJSONObject(JSONStringHUM);
tmp := TJSONObject(ValueTMP).Get(0).JsonValue.Value;
hum := TJSONObject(ValueHUM).Get(0).JsonValue.Value;
fxWidgetLCDLabel1.Caption.Value := StrToFloat(tmp);
fxWidgetLCDLabel2.Caption.Value := StrToFloat(hum);
Finally
//Except {ErrorMsg / RaiseMsg(Const Error:String)}
J.Free;
End;
End;
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
- ③fxSwitch1-OnSwitch事件
點選開關,打開/關閉計時器。
Procedure fxSwitch1OnSwitch(Sender: TObject);
//開啟、關閉溫濕度讀取
Begin
fxTimer1.Enabled := fxSwitch1.IsChecked;
if fxSwitch1.IsChecked = False Then
begin
fxWidgetLCDLabel1.Caption.Value := 0;
fxWidgetLCDLabel2.Caption.Value := 0;
End;
End;
Begin
fxWidgetLCDLabel1.Caption.FillOff.Color := NULL;
fxWidgetLCDLabel2.Caption.FillOff.Color := NULL;
fxWidgetLCDLabel1.Caption.Format := '000.00';
fxWidgetLCDLabel2.Caption.Format := '000.00';
End.
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# 8. 運行結果
使用滑鼠在 PinToo 功能表,點選[儲存至資料庫]
按鈕,將其儲存至資料庫,點選[除錯運行]
確認能夠正常打開。
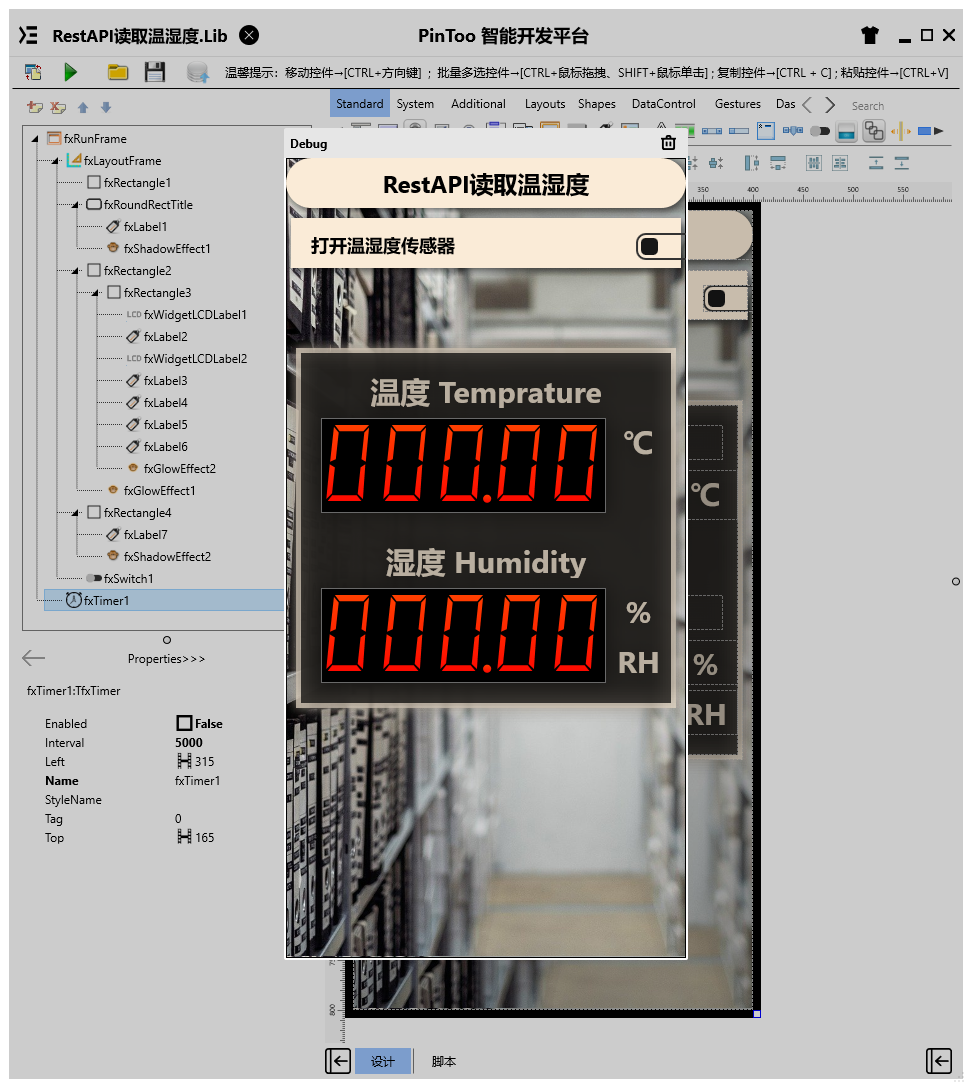
通過同步中心,將程式上傳至手機PinToo運行;同步時,請確保手機已經運行PinToo,並且已經登陸。
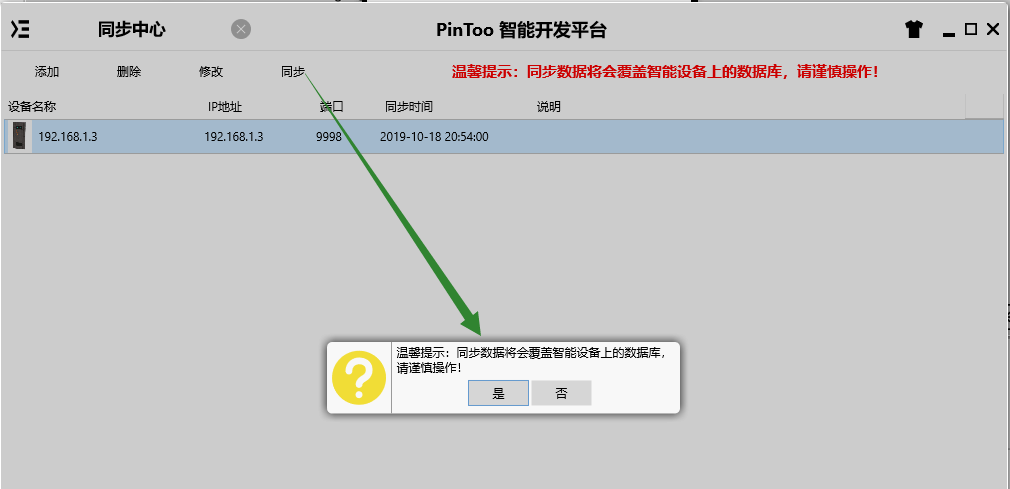
打開開關,程式每隔五秒讀取溫濕度數據並顯示在儀表中。
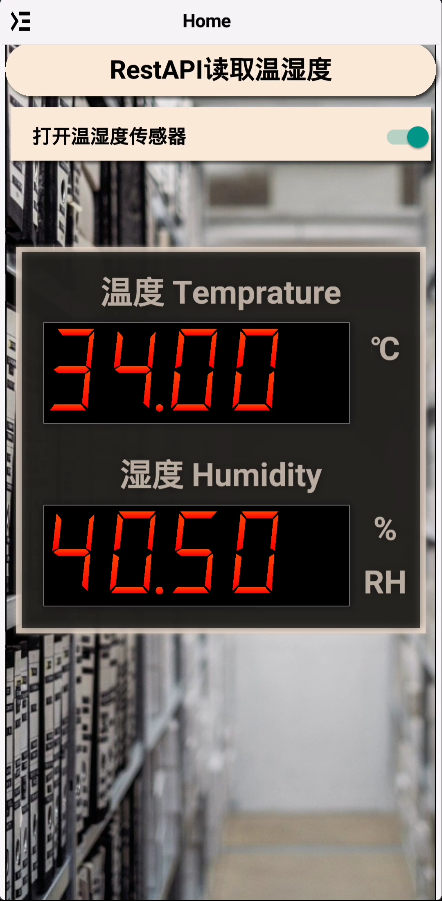